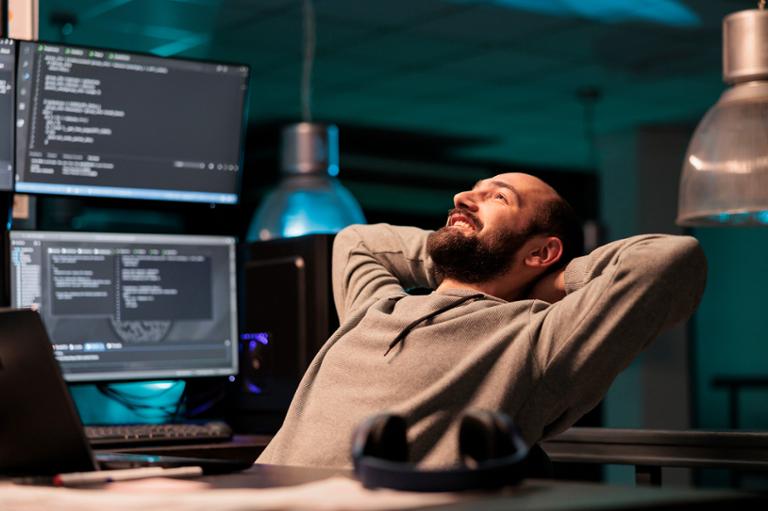
Let’s say you’re a tech professional who’s just finished school. You might think you know everything you need to land your first full-time software development job—but when you read the job postings, you’re hit hard by reality: a lot of organizations want tech pros who know technologies you’ve never even heard of. What do you do now?
This has been an ongoing problem for decades. While Computer Science courses and similar tech-centric programs tend to be great for laying out the foundational material (i.e., the underlying explanations of how software and hardware actually work), they often fail to teach all those “side” technologies that developers are required to know when they actually enter the workforce.
Alternately, these technologies are offered as optional electives, and the students skip the courses, not realizing such knowledge actually is not optional at all.
Before we begin, let’s take a brief look at what technologies you might want to learn (you won’t be able to learn all of these at once, but let’s at least build a list).
- Docker, containerization, and orchestration tools such as Kubernetes
- Bash shell concepts
- Git/GitHub/GitLab, especially branching, pull requests, and general approach to teamwork with git
- CI/CD pipelines
- Clouds (especially AWS, Google Cloud, Azure)
- Serverless architecture
- Agile and Scrum methodologies
- Development technologies such as authentication flows like OAuth and JWT
- NoSQL databases
- UI/UX concepts, such as accessibility
Additionally, there are topics that some universities cover well, while others don’t, such as:
- Debugging tools
- Modern front-end frameworks (Angular, React, Vue)
- JavaScript and TypeScript coding
- Backend development with languages such as node.js and Python
- Full stack architecture and all its little nuances (like CORS issues and so on).
And finally:
- Anything and everything relating to building AI powered apps. (We’re not really talking about simply using AI such as Copilot to help you write your code; we’re talking about building with AI.)
This is quite a list and would overwhelm anybody, leaving them wondering why they even bothered getting the degree (but trust us, you did the right thing by learning the foundational work). Let’s put together a list and a plan for learning.
(Pro Tip: Before we begin, let’s bring up something importat: Of all the above technologies, the single most important one to learn right now is probably Docker. Docker is a fundamental part of software development, yet most programs—from bootcamps to university degrees—rarely cover them. And oddly enough, the second-most-important is probably bash shell techniques. These are critical to cover on your own if no instructor has done it for you.)
Prioritize Your List
This list we created would probably take several years to get through. That means you’re going to want to prioritize it. You have to think about where you want to be say a year from now, three years from now, five years from now, 10 years from now, and so on.
We already gave you the number one item on the list: docker. And number two is bash. Number three is probably git. (The reason we didn’t put git first is most students seem to learn at least the basics of git.) But after that, it’s totally up to you. One thing you might do is categorize them. For example, if you’re interested in doing cloud development, you’re probably also going to be deploying to the cloud—and that means learning the cloud aspects of CI/CD.
If you plan to work for small or startup type companies, you will definitely want to master your Agile skills. You’ll want to learn how to do two-week sprints and daily standups. Startups also typically love NoSQL, especially MongoDB, as well as any and all things cloud.
On the other hand, if you want to go to work for a large corporation, they tend to be a little bit more traditional in their approaches. They might not use Agile as much; they might embrace older waterfall-style approaches to building software.
If you want to study cloud technologies, divide it up into smaller services, such as how to allocate a server in the cloud, how to use storage buckets, and so on. Then consider getting certified. Amazon has an especially good certification track, which can take a few years to complete, so you want to plan to start very early on at the beginning and set out a roadmap that aligns with your broader goals.
Learning How to Learn
Software development consists of lifelong learning. Innovation progresses at such an amazing rate that you can plan on an entirely new generation of technology every seven years or so. And that means having to constantly learn new things. And you’ll have to do this without the help of a university professor or grad students.
Learning on your own requires the following:
- Reading official documentation.
- Going through online tutorial.
- Trying out online examples.
- Getting answers from either stack overflow or ChatGPT.
- Practice the techniques by coding them over and over.
One option is to take online classes; there you will find people to help, but not in the same way as when you were in school. Many classes are not live, meaning you’re watching a pre-recorded video and cannot simply stop the professor and ask questions. Questions take place in online forums. Often these forums are monitored by a few people, but mostly it’s other students who are answering the questions, and the answers may or may not be correct.
Another option is to learn as you go, hopefully with the help of mentors and co-workers who can teach you how things work. While self-learning is laudable, you can (and should) still ask people for advice when you can.
Build Projects… and Blog About It
As you study new technology, practice building projects that use it. Try to make them as “real world” as possible, while keeping them relatively small. As you build them, you will find that your understanding of the technology will expand. The extra benefit is that you can make it part of an online portfolio to demonstrate your work.
You might consider uploading your projects to GitHub and making the repo public. People have a tendency to find repos that are related to what they’re looking for, and they also tend to fork them. This is where it gets interesting: When people fork a project, they usually make changes to it, and you can actually learn from the changes that they make. (You might even want to pull the changes back into your own project.)
Another thing that helps you master the technology is if you write a blog about it. Write the blog as if you’re explaining it to somebody else sitting right in front of you. You’ll find that by “teaching” the material this way, you’ll actually learn more about it yourself.
Join Online Communities
Another way to learn about any new technology is by joining an online community devoted to it. Be careful in your approach here. Start discussions; for example, say something like, “Hey everybody, I’m working on this project and I’m thinking about doing it this way.” Then briefly describe what you’re up to. You might be surprised how people join in and share their thoughts, all without you having asked a single question.
Master the Art of Solving Problems
You probably got your fair share of learning how to problem-solve in school. But like everything else, this is a skill that evolves over time. Problem-solving is something that everybody innately has in them; the key is bringing it out.
1. Embrace the Debugging Mindset:
Systematic Approach:
- Don't jump to conclusions. Break down complex problems into smaller, manageable parts.
- Use a methodical approach like the scientific method: define the problem, hypothesize, experiment, analyze, and conclude.
- Document your process. This creates a valuable record and helps you identify patterns.
- Root Cause Analysis:
- Go beyond fixing symptoms. Dig deep to find the underlying cause. Techniques like the "5 Whys" can be invaluable.
- Don't be afraid to question assumptions.
2. Enhance Your Analytical Toolkit:
Data-Driven Decisions:
- Learn to interpret data and use it to inform your solutions.
- Familiarize yourself with tools for data analysis and visualization.
- Algorithmic Thinking:
- Practice designing and implementing algorithms. This strengthens your logical reasoning.
- Explore different problem-solving paradigms like divide-and-conquer, dynamic programming, and greedy algorithms.
- Learn to read and understand existing code:
- Reading other folks’ code will show you different coding styles, and different methods of solving similar problems.
3. Cultivate Collaboration and Communication:
Pair Programming/Debugging:
- Work with experienced colleagues to observe their problem-solving processes.
- Explain your thought process aloud. This helps clarify your thinking and identify blind spots.
- Knowledge Sharing:
- Participate in code reviews and technical discussions.
- Document your solutions and share them with your team.
- Ask questions, and do not be afraid to say that you do not understand something.
- Clear Communication:
- Articulate problems and solutions clearly and concisely.
- Learn to communicate technical concepts to non-technical stakeholders.
4. Continuous Learning and Exploration:
Stay Updated:
- Technology evolves rapidly. Dedicate time to learning new technologies and methodologies.
- Follow industry blogs, attend conferences, and participate in online communities.
- Personal Projects:
- Work on projects outside of your job to explore new areas and challenge yourself.
- Contribute to open-source projects.
- Embrace Failure:
- View failures as learning opportunities. Analyze what went wrong and use that knowledge to improve.
- Do not be afraid to try new things, and do not be afraid to break things.
5. Leverage Resources and Communities:
Online Forums and Communities:
- Platforms like Stack Overflow, Reddit's r/programming, and GitHub are invaluable resources.
- Don't hesitate to ask for help when you're stuck.
- Mentorship:
- Seek out experienced mentors who can provide guidance and support.
- A mentor can help guide you through complex problems, and provide context to large systems.
- Online Courses and Tutorials:
- Websites such as Coursera, edX, and Udemy offer a wealth of learning resources.
Keep Studying the Foundations
Remember to practice on coding challenge websites. This won’t directly cover any of the big list we made at the top, but it will continue to build your foundations. For a senior developer, it’s very obvious when some code is written very well by somebody with lots of experience. Where does this experience come from? Just plain years of coding combined with practicing code challenges.
Conclusion: Commit
Finally, promise yourself that you will spend at least a couple hours a day practicing and learning new concepts. The key is to commit and practice. And while it may feel like you are only making baby steps, in fact you are learning and improving. Good luck!