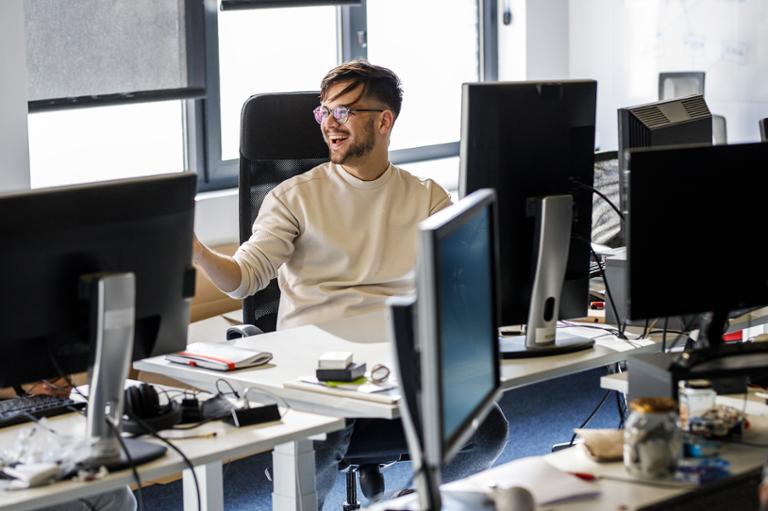
Hiring managers love code challenge websites such as LeetCode, but it’s debatable how useful such sites really are in terms of practicing for the type of coding work you’ll actually find in a job. Most developer jobs focus on building features in software, not optimizing algorithms.
Nonetheless, there’s a lot of utility in exploring LeetCode and its sibling websites, because understanding the nuances of how sophisticated algorithms work is fundamental to good coding skills.
Unfortunately, people who take alternative routes through their careers, such as bootcamps or online learning courses, don’t always get the extensive training needed to solve such coding challenges. This puts them at an extreme disadvantage, especially since many companies outright use LeeCode challenges as part of their hiring processes.
But don’t stress; regardless of whether you survived a Data Structures and Algorithms course at a university or didn’t take any courses anywhere, you can still master the algorithms needed in coding sites such as LeetCode. Here’s the process for tackling them.
If You’re Brand New to Algorithms…
If you’re a newbie to coding challenges, you’ll need to understand a few concepts up front.
- The approach to coding these is a bit different from everyday business coding such as, say, writing CRUD operations for a database.
- While it’s fun to optimize your solutions and make them as fast as possible, this isn’t always the best approach in the “real world.” You need code that’s readable and maintainable long after you’ve moved on from the project.
- Make sure you spend time learning the foundational algorithms: the basics of how sorts work, how linked lists work, and how to use linked lists to build stacks and queues. Understand terminology such as “last in first out” and “first in first out.” (We don’t have space here to teach such things, but there are plenty of blogs and YouTube videos available.)
- Do not let GitHub Copilot and other such AI plugins do the work for you. While it’s useful to see a solution up-front and learn from it, you need to ultimately code these yourself or you’ll never learn. Our recommendation is to disable Copilot while you’re working on these kinds of problems, which leads us to our final tip:
- Until you get really good, don’t code in the tiny little input box in the LeetCode page. Copy the function header into VS Code or whatever IDE you prefer, then take advantage of the syntax highlighting and other features of that IDE. Then when you’re finished, paste the code back into the LeetCode page. (With Python, you might have to adjust the indenting.)
Read the Rules Carefully
It’s way too easy to miss things in the challenge rules. For example, if you’re searching for an item in a list or array, are you expected to return the value or the index? Is there always a solution to every challenge? Do you need to handle the situation where there is no solution? What do you do if there are multiple solutions to a single challenge?
We recommend you take the following approach to reading the rules:
- Skim the rules to get a vague idea of what’s going on, but initially just focus on the inputs and their types. Are you passing an array into the function or individual numbers or strings?
- Read the entire rules again, this time focusing on what the parameters are going to do, but also getting a vague idea of the overall goal of the challenge (such as merging two arrays, finding missing items, and so on).
- Read the rules again—yes, a third time. Make sure you understand everything. You don’t want to miss any details and do the challenge wrong!
Now you should have a full and complete understanding of the problem. Next, look at the examples. Look at the example inputs and the output. Go through each example and make sure you understand why the particular inputs led to a particular output. Does one example not make sense? You might need to re-read the rules yet again.
(When you’re applying for jobs that involve coding challenges, this advice will come in useful, as many companies will create obtuse questions; you may have to read through the materials multiple times to figure out what’s required in terms of output.)
Now unless you have a very clear idea of how to quickly solve the challenge, wait. Don’t attempt it yet. You’re still in the learning phase, which we cover next.
The Approach to Practicing: Learn First
Too often people dive into a LeetCode challenge without really understanding how to code algorithms, and they quickly become frustrated and quit. Instead, your best bet is to start by looking at solutions and seeing how other people did it.
In pretty much every field imaginable, people first observe a mentor doing the work. If you want to become a pilot, you’re not expected to take the controls of a jetliner yourself; it’s a similar situation in pretty much profession, from surgeon to hair stylist.
Our recommendation: Start with some of the LeetCode challenges labeled “easy.” But don’t try them yet; instead, search YouTube for “leetcode” followed by the name of the challenge. Watch the person solve it for you. (If they don’t solve it, or they don’t offer a good explanation of their steps, find another video.) Wait to try the challenge yourself; it’s important to see the approach people take in tackling such problems.
As you watch, make sure you understand each step. Then set the video aside; don’t try to solve it on your own yet. Wait at least a day or so. Then on the next day, see if you can solve the same problem yourself, once you’ve had a chance for the information to hopefully settle in your mind. (Yes, you already watched somebody solve it, but you’re still in the observation phase.) If you get stuck, feel free to watch the video again. Then repeat the process.
Do this same process for several different coding challenges. After a dozen or so challenges, you should have a good feel for the approach needed to solve them.
Now you’re ready to try one on your own, without any mentor-style assistance. Make sure whichever one you select is labeled “easy.” If you get stuck, you can watch a YouTube video for some hints, but stop the video once you have enough to continue on with the problem.
About the Other Users’ Solutions…
In LeetCode, you have access to all the users’ solutions to the challenge for the same programming language you’re using. Treat these with a bit of healthy skepticism. Even though these examples might have passed all the tests, and they might be blindingly fast, don’t automatically assume that what you see is “good code.”
Let’s talk about that for a bit. Most coding languages let you write code in a way that radically condenses dozens of lines of code down to just a few “tight” lines of code. While such code might pass a high “cool factor,” the reality is that such code is usually extremely difficult to read and understand.
And worse, such code is usually nearly impossible to maintain, especially by junior developers. Imagine a scenario: You’ve written some really tight code, and now you’ve been promoted and are moving to a different team within the company. A newbie junior developer takes over where you left off, and is tasked with adding a new feature.
In order to add the feature, the junior dev needs to modify the code you wrote… except your code is nearly impossible to understand. The junior dev doesn’t ask for help because he or she thinks they understand it—but they don’t. They modify it, and it passes the current tests, but would fail in other cases those tests didn’t cover, which the new feature needs. A few weeks or months later, your code crashes; several senior devs come in and it takes them days to track down the problem and fix it.
That’s not a good solutioon, to put it mildly. Great code is clear and obvious.
Have Fun With It
But with the above in mind, it’s still fun to see how much you can tighten up your code, optimize it, and see if you can beat out the fastest solutions. While you wouldn’t normally want to commit such code to a professional repo, there’s value in understanding where bottlenecks are in your current working solution.
It’s also interesting to see whether writing your own loops is faster than the built-in library functions. (For example, in Python, most of the library functions are written in C and compiled to assembly code, meaning they’re very fast. But in other languages like C#, that’s not the case, and the built-in functions might not always be the fastest.)
Moving Up To Medium and Hard Challenges
As you finish the easy challenges, you’ll soon find you’re ready for the medium challenges. At this point, you should be comfortable attacking them yourself without having to search for solutions on YouTube. The goal here is twofold:
- Grow in confidence
- Be able to build good solutions
It’s a big leap from the easy to medium ones, but if you practiced the former enough, you should be pretty comfortable moving up to medium (and eventually the hard ones). The medium ones usually make use of more complex algorithms that might require multiple data structures. You’ll usually have to think multiple steps ahead.
Try a solution out on paper first. Think through different approaches and see which one makes the most sense.
Try breaking it up into smaller problems, if possible... and definitely don’t go for the most optimal solution right away. First, develop a well-written version, even if the code appears a bit on the long side. The goal is to understand the problem and come up with a clear path to solve it.
And don’t be too hard on yourself. If you get stuck, take a break. Do something else and return after you’ve had a chance to clear your head. And if you’re still stuck, then have a peek at some of the existing solutions and try to learn from them.
Conclusion
Taking on coding challenges such as LeetCode is no small task, but it’s well worth it and, arguably, required to make it in the professional coding field. Take the challenges slowly, and over time you’ll find you’re getting better and better at solving them. Then you’ll be well on your way to mastering the tech challenge part of any interview.