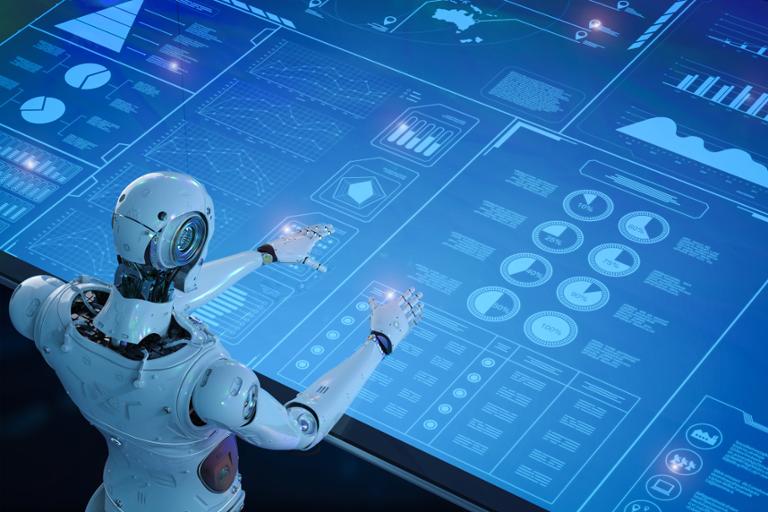
We’ve reached a point in AI advances where almost any app can benefit from having some form of AI embedded in it. Even something as simple as a calculator app could include a chatbot specifically about math, answering questions such as, “What is the formula of a circle?”
Python is the language that the AI world has embraced, and as such, there are many different libraries and frameworks you can include in your app to add AI features. All you need is the good old “pip install” to add them, along with the right hardware. Let’s get the hardware part out of the way right now, and then we’ll look at the concepts and libraries.
To use AI in your app, you need a good GPU; typically, you’ll want a NVidia GPU that supports the CUDA framework. The reason for this is that, while typical CPUs in a computer might have six or eight cores, GPUs typically have a couple thousand cores. They’re specialized, unlike the cores in a CPU. But they work very well for the kind of algorithms needed for AI. If you don’t have a NVidia GPU with CUDA support, you can spin one up on Amazon.
AWS also has a new set of CPUs that are as good as the GPUs. You can learn more about them here.
What Is an AI Model?
Before we begin, let’s give a quick rundown of the very heart of today’s AI: the model, which holds all the data the AI needs. While you could spend months studying how an AI model works and how the data is stored in it, you don’t have to. In fact, that’s a specialty, and not something most developers have time for. Instead, most of us just want to add AI to our app without having to understand what’s under the hood. The important thing to remember is that the model holds the data and can be trained with new information. Most of the time, you’ll install a model that has been pre-trained with data so that you don’t have to train the model first.
There are different types of models, such as Large Language Models (LLMs) that process human language. There are also computer vision models, which can process and generate images. Similarly, there are speech models that can process spoken words, and generate speech.
LLMs digest and process massive amounts of written language, and that’s why tools like ChatGPT are so good at understanding your prompts and writing responses that sound almost human. But as programmers we need to understand that LLMs can be trained with a specific set of information. For example, a calculator app probably doesn’t need an LLM that’s trained with an encyclopedia of medical knowledge. LLMs tend to get very large (think gigabytes, even tens of gigabytes), and so it makes sense to use highly specialized LLMs that are much smaller. Online communities have taken the time to build specialized LLMs that you can use in your app. Some are free; some are premium. The largest online community to find LLMs is a site called HuggingFace.
Pro tip: While we’re on the topic of math, it’s important to realize that LLMs are great at human language, which is what they’re built for. They aren’t good at math. They can, however, be trained to offer explanations of math. So, in the case of a calculator app, you’ll likely want to still build a basic app the traditional way, but also provide a chatbot that can answer questions about math (such as “What is the formula for the area of a circle” or “In mathematics, what’s the difference between an integral and a derivative?”).
What Libraries Should You Start With?
Python has a huge number of frameworks and libraries available for adding AI capabilities to your app. But to get some basic AI going, you only need a few libraries, depending on how deep you want to go into the AI. Here are the libraries to start with:
- Transformers. The place to start is the Transformers library created by Hugging Face. The page for this library has plenty of examples you can draw on. But as you’re looking at those examples, consider taking a free online course put together by Hugging Face. You can find it here.
- PyTorch. Next, you’ll need to learn the library called PyTorch. PyTorch is a Python wrapper around an open-source library called Torch; essentially this means it gives Python programmers access to Torch. PyTorch is a Machine Learning library, with computer vision capabilities and natural language library. Learn as much as you can about PyTorch. This library is essential not only to generative AI, but to machine learning and AI in general. You can find out more about PyTorch here.
- TensorFlow. This is a set of tools and libraries created by Google; they call it an ecosystem for machine learning and deep learning. You can learn more about TensorFlow here, including tutorials here.
Pro tip: When learning these libraries and tools, take them slowly, and work through the entire tutorials. Type in the code yourself and take time to understand what each line of code is doing before moving to the next step in the tutorial.
Also study the ecosystems. PyTorch has its own extensive set of tools called the PyTorch Ecosystem. You can find out more about TensorFlow’s ecosystem from its home page and click the menu called Ecosystem.
Additional Libraries
The above three frameworks form the basis of what you need. But you’ll also need to know additional libraries that aren’t AI-specific, as well as some additional AI libraries. These play a critical role in developing AI apps in Python.
- Pandas and NumPy: Pandas is a general-purpose library for data analysis, especially data that’s in the form of arrays (much like rows and columns of a spreadsheet); Numpy handles computing and linear algebra.
- MatplotLib: This library is for data visualization. It’s easy to use and can produce very nice professional-looking charts.
- SciPy: This is a library for scientific computing.
- Scikit-learn: This is a machine learning library that’s been around for almost two decades but is still updated today and relevant.
- Keras: This is a library for neural networks.
Pro tip: If you start googling for libraries and frameworks, you’ll find a lot of them. Don’t try to learn them all. Start with the essentials, Transformers, PyTorch, TensorFlow, Pandas, NumPy, and MatPlotLib. Get comfortable, and then add in SciPy. From there you can start googling and exploring more, such as the other two we recommend, Scikit-learn, Keras.
Learn How to Use Existing Models
You’ll want to start with existing models that you can find on Hugging Face. Note that these models can be several gigabytes in size, which can present two problems: Download time and storage space. This is another reason you might want to use a GPU on a cloud provider such as AWS, as you can easily spin up a server that has the required space, and downloading from Hugging Face to an AWS instance is quite fast.
One great place to start is OpenAI’s own community LLMs; go to Hugging Face’s model search page and search for openai.
Next, you’ll want to practice writing code that makes use of these existing models without adding additional training to the models. The ideal practice here is a simple chatbot.
Deeper Concepts with Sentence Transformers
Next, you’ll want to practice different concepts with LLMs, such as sentence transformers. A great Python library for that is called just that, Sentence Transformers. This way, you can start to understand how LLMs link together words based on context, and from there make connection between words (such as “feline” and “cat” are often similar in most contexts; this is how you can ask ChatGPT about what foods a “feline eats” or what foods a “cat eats” and it will know you mean essentially the same thing in either case). Look at the examples with this library, as they’re quite simple to get up and running.
In addition, take note of the different LLMs available to this library, as you can choose which one you want. Just make sure you download the appropriate one first to your computer or hosted server.
Training and Fine-Tuning a Model
While many models you can just roll with and not need to add additional training, in some cases you’ll want to fine tune them. This is a huge concept and more than we can cover here. But it essentially means adding new information to the model. For example, if the model is about current news, the news would effectively end the day the model was trained. But news keeps happening, and such a model would need to be fed additional news stories, so it remains relevant.
The Sentence Transformers library we just mentioned has fine tuning features which you can use from your python apps. You can learn about it here.
Want to Pre-Train a Model?
Most work with LLMs and other AI models involves starting with a pre-trained model (often found on Hugging Face). You download the model you want to start with, and then go from there. You can continue to train the model, a step called fine-tuning.
But pre-training a model requires a different set of skills, as well as huge compute resources and lots of time; we’re talking months to train a medium-sized LLM. We’re not going to go into detail here, because, frankly, it also requires a lot of knowledge and skills, more than we could possibly cover here. If you’re interested in going this route, you’ll need to learn as much as you can about LLMs, as well as completely mastering the libraries such as PyTorch. Read the original paper about Generative AI called Attention is All You Need, as well as an important paper called "BERT: Pre-training of Deep Bidirectional Transformers for Language Understanding.”
Pro tip: As a Python developer interested in learning about AI, you probably don’t want to start with pre-training concepts. First start with learning how to use LLMs and other AI models in your apps. Then explore ways to fine-tune LLMs. Then after you fully understand fine tuning, start to learn about pre-training. But don’t get dismayed! If you’re serious about learning it, you can. It just takes time and requires more than what we can offer here.
Conclusion
As with learning any technology, take it slowly and plan to practice. AI in particular takes time to grasp the concepts alone, much less practice the coding. So, divide up your time between concepts and coding. The concepts are especially important here because it’s easy to learn how to snap in some libraries and add a chatbot to an app through a minimal amount of coding; but learning the actual concepts will further your career and help you to truly become an AI expert and land better jobs as you move forward.